Learn how you can change the pricing display so it’s easier for customers to compare unit price across products.
If several products will have different unit prices, you’d need a more advanced way to do this, but here are a couple methods to get you started with adjusting the WooCommerce price display.
For either method, there are a couple important filters we’ll need to use:
woocommerce_get_price_html
: changes the way price is shown on the product and shop pageswoocommerce_cart_item_price
: changes the way product prices are shown in the cart table (not at checkout since only quantity / total price are shown here, not the unit price).
Filter WooCommerce Price Display for All Products
Let’s say you want to add a label to the end of all of your product prices, such as “per package” (helpful if your products are bundles of something or come in pre-packaged sets). You can change the pricing display to append this label to the price with the product and cart price filters:
<?php // only copy opening php tag if needed // Adds "per package" after each product price throughout the shop function sv_change_product_price_display( $price ) { $price .= ' per package'; return $price; } add_filter( 'woocommerce_get_price_html', 'sv_change_product_price_display' ); add_filter( 'woocommerce_cart_item_price', 'sv_change_product_price_display' );
The woocommerce_get_price_html
filter will change this on your shop pages:
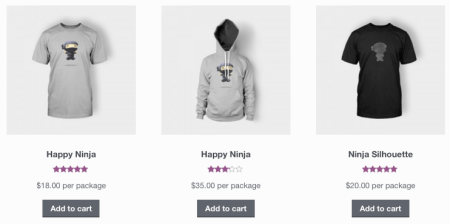
and on your single product pages:
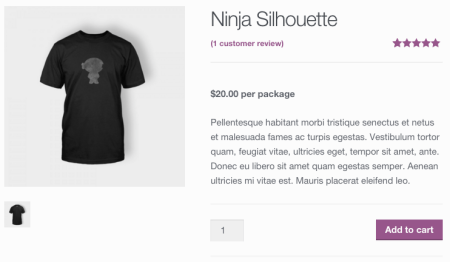
The woocommerce_cart_item_price
filter will adjust this display within the cart as well:

Filter WooCommerce Price Display for a Single Product
What if you want to specifically change the price display for a particular product? This can be done with the same filters, as they’ll also pass additional arguments for you to conditionally change the price. For example, let’s return to our skewer example from the question. While skewers are sold individually at $2 per skewer, we want to show “$15 per kg” as the price instead so customers can compare different products.
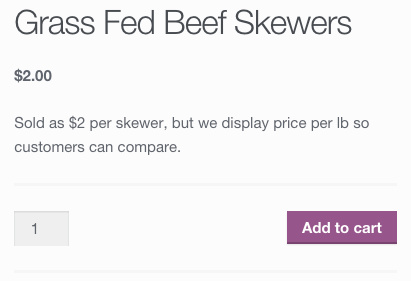
This time we’ll separate out these filters and use two functions to modify the product and cart prices. We’ll first check for the product ID, then change the pricing display for that product alone.
function sv_change_product_html( $price_html, $product ) {
if ( 337 === $product->id ) {
$price_html = '<span class="amount">$15.00 per kg</span>';
}
return $price_html;
}
add_filter( 'woocommerce_get_price_html', 'sv_change_product_html', 10, 2 );
function sv_change_product_price_cart( $price, $cart_item, $cart_item_key ) {
if ( 337 === $cart_item['product_id'] ) {
$price = '$15.00 per kg<br>(7-8 skewers per kg)';
}
return $price;
}
add_filter( 'woocommerce_cart_item_price', 'sv_change_product_price_cart', 10, 3 );
The first function will change my product page:
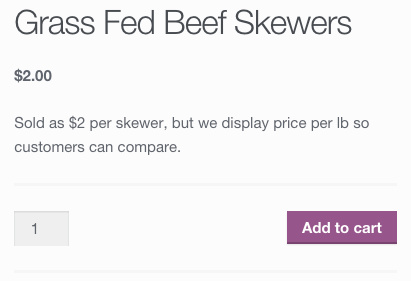
Along with the shop display:
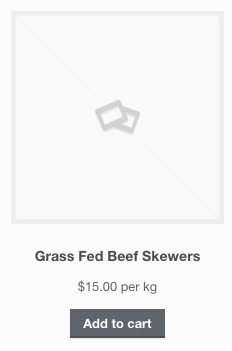
While the second function will adjust my cart display with my new pricing:
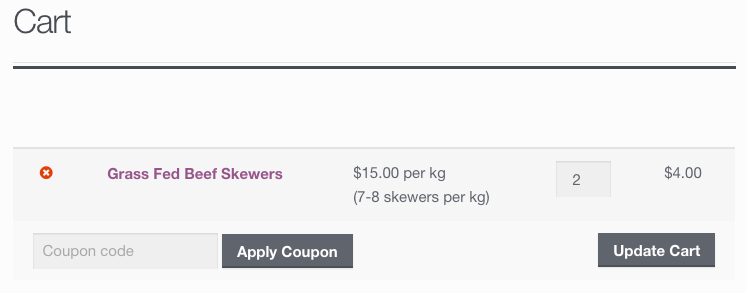
However, if you have multiple products with unit prices, this method will be very cumbersome, and we probably don’t want to have a switch / if statements for every product ID. Instead, I’d recommend using a product custom field to adjust the price display. We can use the ID to get this field, then save ourselves a lot of hard-coding since we’ll pull the field in programmatically.
Filter WooCommerce Price Display Based on Product Fields
If you have to change the pricing display for some products but not all of them, or each product would have a different unit price, using a custom field will give us a far simpler code snippet to implement, as we can add the field for each product, then just retrieve this field with our snippet.
You can add a unit_price
custom field to each product that requires it, then add the unit price value here.
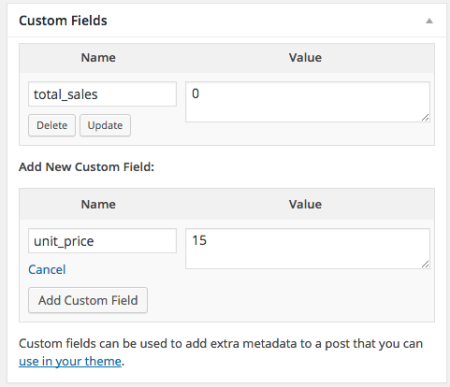
Add custom field
Once you’ve added this, you can then retrieve the unit price, and if one is set for the product, adjust the pricing display to use that unit price and your custom label instead:
<?php
// only copy the opening php tag if needed
// Change the shop / product prices if a unit_price is set
function sv_change_product_html( $price_html, $product ) {
$unit_price = get_post_meta( $product->id, 'unit_price', true );
if ( ! empty( $unit_price ) ) {
$price_html = '<span class="amount">' . wc_price( $unit_price ) . ' per kg</span>';
}
return $price_html;
}
add_filter( 'woocommerce_get_price_html', 'sv_change_product_html', 10, 2 );
// Change the cart prices if a unit_price is set
function sv_change_product_price_cart( $price, $cart_item, $cart_item_key ) {
$unit_price = get_post_meta( $cart_item['product_id'], 'unit_price', true );
if ( ! empty( $unit_price ) ) {
$price = wc_price( $unit_price ) . ' per kg';
}
return $price;
}
add_filter( 'woocommerce_cart_item_price', 'sv_change_product_price_cart', 10, 3 );
(wc_price()
is a handy little function to format numbers with the shop pricing display settings, use it!)
In this case, pricing display on the product / shop page will only be changed if a unit price is set. If not, they price will remain the same:
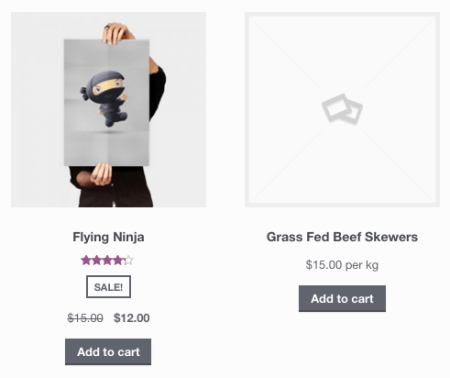
The same will happen with items in the cart — the WooCommerce price display will be conditionally adjusted depending on whether the item has a unit_price set or not in the custom field:
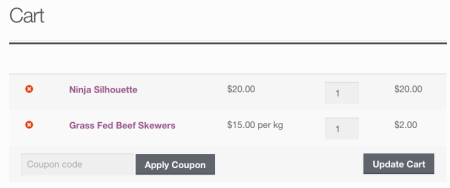
Just because you price items in one way doesn’t mean you need to display that price to customers. Sometimes changing the price display can give customers more information about how the product is packaged, or it can allow customers to more easily compare products.